일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 | 31 |
- 루나네스
- UX베이커리
- 윈도우모바일
- 황광진
- windows mobile 6.5
- 옴니아2
- 윈모데브
- 신석현
- 윈도우폰7
- 마이크로소프트
- 헤이맨
- 데브피아
- MIX10
- 신동혁
- 소년포비
- 윈도우 모바일
- 김춘배
- winmodev
- 훈스닷넷
- 지승욱
- 스마트폰
- 윈도데브
- 쉐어포인트코리아
- 거제도
- 윈도우폰
- 소년포비소프트
- 안드로이드
- 실버라이트 코리아
- 서진호
- 주신영
- Today
- Total
소년포비의 세계정복!!
[C#]급여 계산 본문
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
public class Pay
{
public int EmployeeNumber { get; set; } // 사원 번호
public int SalaryNumber { get; set; } // 급
public int SalaryClass { get; set; } // 호
public int Allowance { get; set; } // 수당
public int TaxAmountPayable { get; set; } // 지급액
public int Tax { get; set; } // 세금
public int CalculatingTable { get; set; } // 급호봉 계산표
public double TaxRate { get; set; } // 세율
public int MediPay { get; set; } // 조정액
public int ResultPay { get; set; } // 차인지급액
public Pay()
{
// Empty
}
}
public class 급여처리
{
public static void Main(string[] args)
{
Console.Title = "급여 처리 프로그램";
//[1] Input
List<Pay> lst = new List<Pay>(); // 입력데이터, 들어오는 만큼 데이터 저장
Pay pi;
string temp1 =""; // 사번
string temp2 =""; // 급
string temp3 =""; // 호
string temp4 =""; // 수당
string btn = "n";
do
{
pi = new Pay();
Console.Write("사원번호 : _\b");
temp1 = Console.ReadLine();
if (Convert.ToInt32(temp1) >= 1 && Convert.ToInt32(temp1) <= 9)
{
pi.EmployeeNumber = Convert.ToInt32(temp1);
}
else
{
Console.WriteLine("사원 번호는 정수 1자리로 입력하세요");
return;
}
Console.Write("급 : _\b");
temp2 = Console.ReadLine();
if (Convert.ToInt32(temp2) == 1 || Convert.ToInt32(temp2) == 2)
{
pi.SalaryNumber = Convert.ToInt32(temp2);
}
else
{
Console.WriteLine("급 입력은 1급 또는 2급만 입력하세요");
}
Console.Write("호 : _\b");
temp3 = Console.ReadLine();
if (Convert.ToInt32(temp3) >= 1 && Convert.ToInt32(temp3) <= 5)
{
pi.SalaryClass = Convert.ToInt32(temp3);
}
else
{
Console.WriteLine("호 입력은 1~5 사이로 입력하세요");
}
Console.Write("수당 : _____\b\b\b\b\b");
temp4 = Console.ReadLine();
if (Convert.ToInt32(temp4) >= 1000 && Convert.ToInt32(temp4) <= 50000 && Convert.ToInt32(temp4) % 1000 == 0)
{
pi.Allowance = Convert.ToInt32(temp4);
}
else
{
Console.WriteLine("수당은 1,000 ~ 50,000 범위의 정수값 중 1,000의 배수로 입력하세요");
}
//[2] Process
// 급호봉 계산표 급과 호에 해당하는 금액
if (pi.SalaryNumber == 1)
{
switch (pi.SalaryClass)
{
case 1: pi.CalculatingTable = 95000; break;
case 2: pi.CalculatingTable = 92000; break;
case 3: pi.CalculatingTable = 89000; break;
case 4: pi.CalculatingTable = 86000; break;
case 5: pi.CalculatingTable = 83000; break;
default: break;
}
}
else if (pi.SalaryNumber == 2)
{
switch (pi.SalaryClass)
{
case 1: pi.CalculatingTable = 80000; break;
case 2: pi.CalculatingTable = 75000; break;
case 3: pi.CalculatingTable = 70000; break;
case 4: pi.CalculatingTable = 65000; break;
case 5: pi.CalculatingTable = 60000; break;
default: break;
}
}
// 지급액 계산
pi.TaxAmountPayable = pi.CalculatingTable + pi.Allowance;
// 지급액에 따른 세율과 조정액
if (pi.TaxAmountPayable < 70000)
{
pi.TaxRate = 0.0;
pi.MediPay = 0;
}
else if (pi.TaxAmountPayable >= 70000 && pi.TaxAmountPayable <=79999)
{
pi.TaxRate = 0.005;
pi.MediPay = 300;
}
else if (pi.TaxAmountPayable >= 80000 && pi.TaxAmountPayable <= 89999)
{
pi.TaxRate = 0.007;
pi.MediPay = 500;
}
else if (pi.TaxAmountPayable >= 90000)
{
pi.TaxRate = 0.012;
pi.MediPay = 1000;
}
// 세금 구하기
pi.Tax = (int)((double)pi.TaxAmountPayable * pi.TaxRate) - pi.MediPay;
// 차인지급액 구하기
pi.ResultPay = pi.TaxAmountPayable - pi.Tax;
lst.Add(pi); // 컬렉션에 추가
Console.WriteLine("입력(y), 종료(n) :");
btn = Console.ReadLine().ToLower(); // 소문자로
} while (btn == "y" && lst.Count <= 4);
Console.Clear();
//[3] Output
IEnumerable<Pay> q = from p in lst orderby p.EmployeeNumber select p;
Console.WriteLine("사번 급 호 수당 지급액 세금 차인지급액");
foreach (var item in q)
{
Console.WriteLine("{0}\t{1}\t{2}\t{3}\t{4}\t{5}\t{6}", item.EmployeeNumber, item.SalaryNumber, item.SalaryClass,
item.Allowance, item.TaxAmountPayable, item.Tax, item.ResultPay);
}
}
}
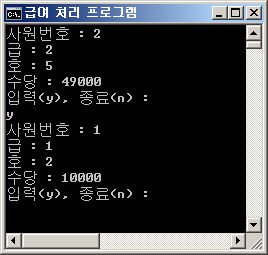
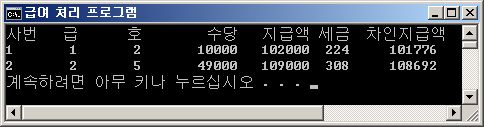
'프로그램 세상 > C#' 카테고리의 다른 글
[C#] 람다식(Lambda Expression) (0) | 2009.10.22 |
---|---|
[C#]Visual Studio 2008에서 DLL 만들고 사용하기 (0) | 2009.10.22 |
[C#] 체중 관리 프로그램 (0) | 2009.10.22 |
[C#] 외부 응용프로그램 실행 (0) | 2009.10.22 |
[C#] 세가지 Timer 와 그 차이점 (0) | 2009.10.14 |